【VS Code開発】効率的なAPI設計-Swagger編
いちいちswagger editorを開いて、コードを編集して、見るというのは結構めんどくさい。
そこで、vscode上で、swagger editorのような役割をしてくれる vscodeの拡張機能を紹介します。
目次
VS Codeの拡張機能で開発スピード爆速化するために
こちらを導入することで、vscode上でAPIを見ることができます。
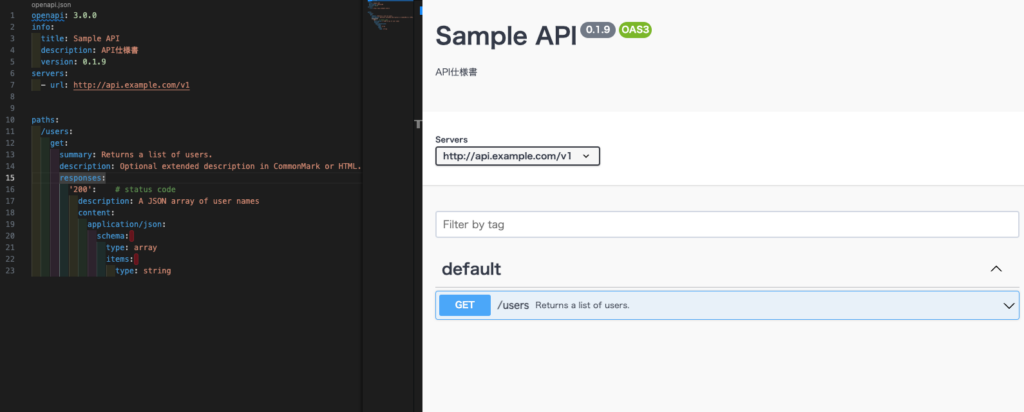
swaggerの書き方
導入
導入部は、公式に従って、以下のように書きます。(以下のコードはサンプルコードです。適宜編集してください)
openapi: 3.0.0
info:
title: Sample API
description: API仕様書
version: 0.1.9
servers:
- url: http://api.example.com/v1
API記述
apiの記述方法は、公式に従って書きます。
まず、paths:を記述します。次に、getメソッドの/usersを記述 以下はその例です。
paths:
/users:
get:
summary: Returns a list of users.
description: Optional extended description in CommonMark or HTML.
responses:
'200': # status code
description: A JSON array of user names
content:
application/json:
schema:
type: array
items:
type: string
他のメソッドにしたい場合
getと記述されていた箇所をpostなどに変更するだけです。
/users:
post:
summary: Returns a list of users.
description: Optional extended description in CommonMark or HTML.
responses:
'200': # status code
description: A JSON array of user names
content:
application/json:
schema:
type: array
items:
type: string
パラメータを導入したい場合
paths:
/users/{userId}:
get:
summary: Returns a user by ID.
parameters:
- name: userId
in: path
required: true
description: Parameter description in CommonMark or HTML.
schema:
type : integer
format: int64
minimum: 1
responses:
'200':
description: OK
リクエストbodyを入れたい場合
paths:
/users:
post:
summary: Creates a user.
requestBody:
required: true
content:
application/json:
schema:
type: object
properties:
username:
type: string
responses:
'201':
description: Created
レスポンスを入れたい場合
paths:
/users/{userId}:
get:
summary: Returns a user by ID.
parameters:
- name: userId
in: path
required: true
description: The ID of the user to return.
schema:
type: integer
format: int64
minimum: 1
responses:
'200':
description: A user object.
content:
application/json:
schema:
type: object
properties:
id:
type: integer
format: int64
example: 4
name:
type: string
example: Jessica Smith
'400':
description: The specified user ID is invalid (not a number).
'404':
description: A user with the specified ID was not found.
default:
description: Unexpected error
同じ記述があるので、コンポーネント化したい場合
paths:
/users/{userId}:
get:
summary: Returns a user by ID.
parameters:
- in: path
name: userId
required: true
schema:
type: integer
format: int64
minimum: 1
responses:
'200':
description: OK
content:
application/json:
schema:
$ref: '#/components/schemas/User' # <-------
/users:
post:
summary: Creates a new user.
requestBody:
required: true
content:
application/json:
schema:
$ref: '#/components/schemas/User' # <-------
responses:
'201':
description: Created
components:
schemas:
User:
type: object
properties:
id:
type: integer
example: 4
name:
type: string
example: Arthur Dent
# Both properties are required
required:
- id
- name